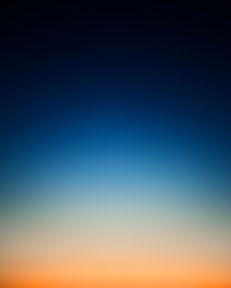


Maewyn's Things
Play "Star Season" Now on itch.io!

Basic Clock Timer
//Countdown seconds by delta_time's microseconds
totalSeconds -= (delta_time/1000000);
var t,years,days,hours,minutes,seconds,timerDisplay;
//Integer Math
t = (60*60*24*365); years = (totalSeconds div t); //Does not handle leap years
t = (60*60*24); days = (totalSeconds div t) % 365;
t = (60*60); hours = (totalSeconds div t) % 24;
t = (60); minutes = (totalSeconds div t) % 60;
t = (1); seconds = (totalSeconds div t) % 60;
//Convert Integers to String Values
years = string(years);
days = string(days);
if (hours < 10) hours = "0"+string(hours); //If timer value is less than 10
else hours = string(hours); // add "0" for two-digit counter
if (minutes < 10) minutes = "0"+string(minutes);
else minutes = string(minutes);
if (seconds < 10) seconds = "0"+string(seconds);
else seconds = string(seconds);
timerDisplay = years + "years / " +
days + "days\n" +
hours + ":" + minutes + ":" + seconds;
draw_text(x,y,"Remaining Time:\n" + timerDisplay);
Measure Variable Range from 0 to 1
var _minVar = -6,
_maxVar = 7,
_var = clamp(score,_minVar,_maxVar),
_var0to1= (_var - _minVar) * (1/(_maxVar - _minVar));
score = _var;
image_blend = merge_color(c_lime,c_red,_var0to1);
draw_set();
/// @description draw_set(font,halign,valign,color,alpha)
/// @param font
/// @param halign
/// @param valign
/// @param color
/// @param alpha
draw_set_font(argument0);
draw_set_halign(argument1);
draw_set_valign(argument2);
draw_set_color(argument3);
draw_set_alpha(argument4);